Have you ever felt like you’re playing a game of whack-a-mole every time an unexpected error pops up in your Lightning Web Component (LWC) code? Or maybe you’re simply looking for ways to make your LWC development process smoother and more efficient. Either way, this is in-depth guide on Advanced Error Handling in Lightning Web Components(LWC) is here to help.
Why Do We Need Advanced Error Handling in Lightning Web Components(LWC)?
Imagine this – you’ve been working on an exciting new feature, but when you deploy the code, you’re hit with a barrage of error messages. Even worse, the error messages are unclear or not properly formatted, making them more of a headache to decode. This is where the power of advanced error handling comes into play.
Progressing from basic error handling to a more sophisticated level allows you to:
- Predict and pre-empt potential issues,
- Keep your application robust and reliable,
- Improve user experience and
- Make debugging a less daunting task.
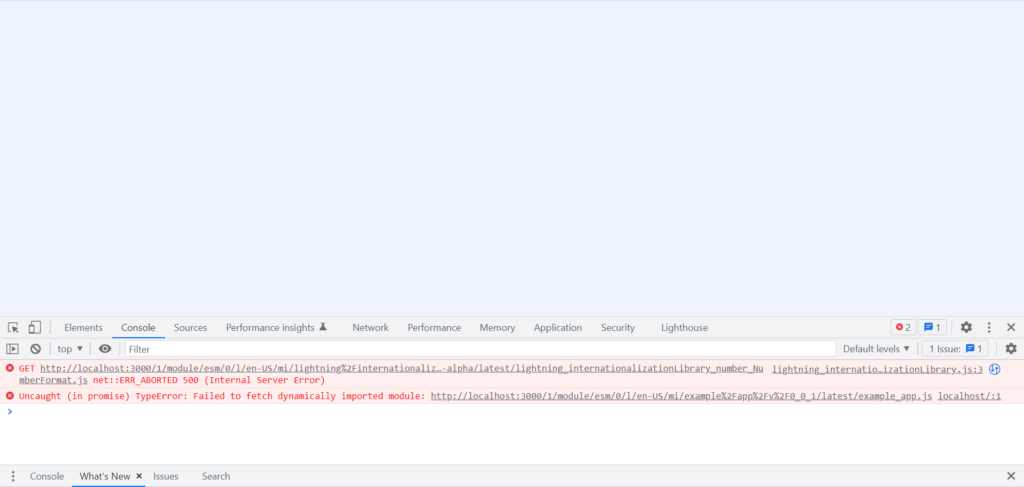
Exploring the LWC Error Handling Playground
Before jumping into the nitty-gritty details of advanced error handling in LWC, it’s essential to have a fundamental understanding of generic JavaScript error handling. Errors in JavaScript usually fall into one of these categories:
- ReferenceError: This type of error occurs when you try to use a variable that doesn’t exist.
- TypeError: This happens when a value is not of the expected type.
- RangeError: This error occurs when a numeric variable is outside its allowed range.
- SyntaxError: This type of error is self-explanatory—it happens when you’ve made a mistake in the syntax of your JavaScript code.
When errors tend to occur in JavaScript, proper use of try...catch
and finally
blocks can come in handy. A try
block encapsulates the code that could potentially trigger an error. When an error is thrown, the catch
block is initiated, allowing the program to respond to the error rather than just crashing. Lastly, the finally
block occurs after the try
and catch
blocks and will execute regardless of whether an error was thrown or not.
Let’s see these concepts in action:
try { // Some code... } catch(error) { console.error('An error occurred:', error); } finally { console.log('This will always run'); }
Armed with this basic understanding of JavaScript error handling, we’re now equipped to delve deeper into the world of advanced error handling techniques in Lightning Web Components.
Base Lightning LWC Error Handlers
LWC introduces a set of base lightning components aimed at making error handling with LWC simpler. The base lightning components extend the basic try-catch error handling principle, enhancing it with better error tracking and user interface notifications. They include the following:
- lightning:notificationsLibrary (AURA): This library provides the user with customized notifications whenever certain actions or errors occur.
- lightning:card: The ‘onerror’ event can be handled within the component and displayed on the card.
- lightning:input: When a user inputs data, you can employ various error checking techniques beforehand to validate the data.
Implementing Async Error Handling
Asynchronous programming is a crucial aspect of Lightning Web Components, especially when dealing with server-side operations. Considering the async nature of promise-based operations, error handling for these cases requires particular attention. Instead of just wrapping a block of code within a try-catch statement, async error handling involves chaining a .catch
method to your promise.
myPromiseBasedFunction() .then(result => { // Handle the result }) .catch(error => { console.error(`An error occurred: ${error}`); });
The .catch
block catches any error that arises during the promise execution or within the preceding .then
block(s).
Uncaught Errors with Error Boundaries
Error boundaries provide an additional safety net for errors uncaught by other error handling methods in our components. In LWC, The imperative wire adapter service provides an error boundary mechanism that prevents the application from crashing.
Creating an error boundary involves wrapping a component in an error boundary parent component that uses the @wire
service to catch and handle errors.
import { LightningElement, wire } from 'lwc'; import { createMessageContext, releaseMessageContext, APPLICATION_SCOPE } from 'lightning/messageService'; import SAMPLEMC from '@salesforce/messageChannel/LWC_Error_Boundary__c'; class ErrorBoundary extends LightningElement { @wire(createMessageContext) messageContext; connectedCallback() { this.context = createMessageContext(); } disconnectedCallback() { releaseMessageContext(this.context); } handleWireError({error}) { this.publishError(error); } publishError(error) { publish(this.context, SAMPLEMC, { errorType: 'UNEXPECTED', message: error.message }); } } export default ErrorBoundary;
Adequate Testing: The Golden Rule of Advanced Error Handling
No discussion on error handling in LWC – or any programming habitat for that matter – is complete without mentioning the central role of testing diligently. Testing frameworks such as Jest come to the rescue by providing a convenient means of simulating potential error scenarios and ensuring our error handling mechanisms duly cover them.
For instance, using Jest, we can simulate an error response from a server and test how our component handles it. Remember, each new error handling technique that you implement in your LWC deserves its corresponding testing routine.
Here are some advanced error handling techniques you can apply in LWC:
- Logging Errors:
Implementing robust logging is crucial for understanding and debugging issues. Use theconsole.error
method to log errors in the browser console. You can include additional information such as the error message, stack trace, and relevant context.
try { // Your code that might throw an error } catch (error) { console.error('An error occurred:', error.message, '\nStack trace:', error.stack); }
- Global Error Handling:
Set up a global error handler to capture unhandled exceptions within your LWC components. This ensures that errors won’t go unnoticed.
window.addEventListener('error', function (event) { console.error('Unhandled error:', event.error); // Additional actions, such as sending error reports to a server });
- Displaying User-Friendly Error Messages:
Provide clear and user-friendly error messages when interacting with components. Use conditional rendering to display error messages based on certain conditions.
<!-- Example in LWC HTML file --> <template> <lightning-card title="Error Handling Example"> <template if:true={hasError}> <p class="error-message">An error occurred: {errorMessage}</p> </template> <!-- Rest of your component markup --> </lightning-card> </template>
// Example in LWC JS file import { LightningElement, track } from 'lwc'; export default class ErrorHandlingExample extends LightningElement { @track hasError = false; @track errorMessage; connectedCallback() { try { // Your component logic } catch (error) { this.handleError(error); } } handleError(error) { this.hasError = true; this.errorMessage = error.message; console.error('An error occurred:', error.message, '\nStack trace:', error.stack); } }
- Using Custom Events for Communication:
Implement custom events to communicate errors between child and parent components. This allows child components to notify their parent components about errors, and the parent can take appropriate action.
// Child Component const errorEvent = new CustomEvent('error', { detail: { message: 'An error occurred in the child component' }, }); this.dispatchEvent(errorEvent);
<!-- Parent Component --> <template> <c-child-component onerror={handleChildError}></c-child-component> </template>
import { LightningElement } from 'lwc'; export default class ParentComponent extends LightningElement { handleChildError(event) { const errorMessage = event.detail.message; // Handle the error in the parent component } }
- Handling Promises and Asynchronous Operations:
When dealing with asynchronous operations, handle promises and usetry...catch
blocks appropriately. This helps in catching errors that occur during asynchronous tasks.
async someAsyncOperation() { try { // Asynchronous code that might throw an error const result = await someAsyncFunction(); } catch (error) { console.error('An error occurred during the asynchronous operation:', error.message); } }
By applying these advanced error handling techniques, you can enhance the reliability and user experience of your Lightning Web Components. It’s important to tailor your error handling strategy to the specific requirements and constraints of your application.
Reference: Error Handling Best Practices for Lightning Web Components