Introducing the topic of Handling Large Datasets in Lightning Web Components may seem a bit at first. As a developer, you might often find yourself dealing with hefty amounts of data, which can prove a challenging task. But fear not, this post aims to lighten your burden and guide your steps to an efficient and sanitized data management process within the Salesforce ecosystem.
Here are some best practices and considerations for dealing with large datasets in LWC:
- Server-Side Pagination:
- Implement server-side pagination to load only a subset of data initially.
- Use Apex controllers to handle server-side logic for fetching paginated data.
Create an Apex controller:
public with sharing class PaginationController { private static final Integer PAGE_SIZE = 10; @AuraEnabled(cacheable=true) public static List<CustomObject__c> getPaginatedRecords(Integer pageNumber) { Integer startIndex = (pageNumber - 1) * PAGE_SIZE; return [SELECT Id, Name, Field1__c, Field2__c FROM CustomObject__c ORDER BY CreatedDate DESC LIMIT :PAGE_SIZE OFFSET :startIndex]; } }
- Create a Lightning Web Component:
paginationComponent.html:
<template> <div class="container"> <template if:true={paginatedRecords}> <ul> <template for:each={paginatedRecords} for:item="record"> <li key={record.Id}>{record.Name}</li> </template> </ul> <div class="pagination"> <lightning-button label="Previous" onclick={handlePrevious} disabled={pageNumber === 1}></lightning-button> <span>{pageNumber}</span> <lightning-button label="Next" onclick={handleNext} disabled={paginatedRecords.length < PAGE_SIZE}></lightning-button> </div> </template> <template if:false={paginatedRecords}> <p>No records found.</p> </template> </div> </template>
paginationComponent.js:
import { LightningElement, track } from 'lwc'; import getPaginatedRecords from '@salesforce/apex/PaginationController.getPaginatedRecords'; const PAGE_SIZE = 10; export default class PaginationComponent extends LightningElement { @track pageNumber = 1; @track paginatedRecords; connectedCallback() { this.loadRecords(); } loadRecords() { getPaginatedRecords({ pageNumber: this.pageNumber }) .then(result => { this.paginatedRecords = result; }) .catch(error => { console.error('Error fetching records', error); }); } handlePrevious() { if (this.pageNumber > 1) { this.pageNumber -= 1; this.loadRecords(); } } handleNext() { this.pageNumber += 1; this.loadRecords(); } }
This example Lightning Web Component (PaginationComponent
) displays a list of paginated records with “Previous” and “Next” buttons. The getPaginatedRecords
Apex method is called to fetch the records based on the page number. The page number is incremented or decremented based on the button clicked, and the records are reloaded accordingly.
- Lazy Loading:
- Employ lazy loading techniques to load data only when needed. For example, load additional data as the user scrolls down the page.
- Use the
Intersection Observer API
to detect when an element enters the viewport and trigger the data fetching accordingly.
The Intersection Observer API is a powerful tool for efficiently detecting when an element comes into or goes out of the browser’s viewport. In the context of lazy loading in Lightning Web Components (LWC), you can use the Intersection Observer API to load a component or its resources when it becomes visible on the screen. Here’s how you can implement lazy loading using the Intersection Observer API:
- Create the Child Component (
lazyChild
):lazyChild.html
:
<!-- lazyChild.html --> <template> <div> <p>This is the lazy child component!</p> </div> </template>
lazyChild.js
:
// lazyChild.js import { LightningElement } from 'lwc'; export default class LazyChild extends LightningElement {}
- Create the Parent Component (
lazyParent
):lazyParent.html
:
<!-- lazyParent.html --> <template> <div> <!-- Placeholder div for Intersection Observer --> <div class="placeholder" lwc:dom="manual"></div> <!-- The actual lazyChild component will be rendered here --> <template if:true={shouldRenderChild}> <c-lazy-child></c-lazy-child> </template> </div> </template>
lazyParent.js
:
// lazyParent.js import { LightningElement, track } from 'lwc'; export default class LazyParent extends LightningElement { @track shouldRenderChild = false; connectedCallback() { // Initialize Intersection Observer when the component is connected to the DOM this.initIntersectionObserver(); } initIntersectionObserver() { const placeholder = this.template.querySelector('.placeholder'); const observer = new IntersectionObserver(this.handleIntersection.bind(this), { root: null, // Use the viewport as the root rootMargin: '0px', // No margin threshold: 0.5 // Trigger when 50% of the placeholder is visible }); observer.observe(placeholder); } handleIntersection(entries) { entries.forEach(entry => { if (entry.isIntersecting) { // If the placeholder is intersecting with the viewport, render the child component this.shouldRenderChild = true; } }); } }
- Use the Components in a Lightning App (
lazyApp
):lazyApp.html
:
<!-- lazyApp.html --> <template> <!-- lazyParent is the entry point, and it uses Intersection Observer to lazy load lazyChild --> <c-lazy-parent></c-lazy-parent> </template>
lazyApp.js
:
// lazyApp.js import { LightningElement } from 'lwc'; export default class LazyApp extends LightningElement {}
- Wire Up the Components: Ensure that you have the appropriate metadata files (
lazyParent.js-meta.xml
,lazyChild.js-meta.xml
,lazyApp.js-meta.xml
) with the correct configurations. For example,lazyParent.js-meta.xml
:
<!-- lazyParent.js-meta.xml --> <?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="lazyParent"> <apiVersion>52.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__AppPage</target> <target>lightning__RecordPage</target> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
- Deploy and Use: Deploy your components to your Salesforce environment, and then you can use the
lazyApp
component in your Lightning App Builder, Record Page, or Home Page. ThelazyChild
component will be loaded when it comes into the viewport due to the Intersection Observer.
This example demonstrates lazy loading using the Intersection Observer API in LWC. The child component is loaded when it becomes visible on the screen, helping to optimize the performance of your LWC applications.
- Use Lightning Data Service (LDS):
- Utilize LDS to cache and manage data on the client side.
- LDS provides a local cache that can be used to minimize server requests for data that has already been fetched.
Lightning Data Service (LDS) is a powerful Salesforce feature that allows you to create Lightning components without writing server-side controller logic. LDS provides access to Salesforce data and metadata, making it easier to build Lightning components that are both powerful and efficient.
Here’s a simple example of using Lightning Data Service in a Lightning Web Component (LWC) to display information about a specific record.
- Create an Apex Class: Create an Apex class to handle the data retrieval. This class should implement the
force:recordData
interface.
// ExampleController.cls public with sharing class ExampleController { @AuraEnabled(cacheable=true) public static String getRecordId(String recordId) { return recordId; } }
- Create the Lightning Web Component (
ldsExample
):ldsExample.html
:
<!-- ldsExample.html --> <template> <lightning-card title="Record Details" icon-name="standard:account"> <div class="slds-m-around_medium"> <template if:true={record.data}> <div class="slds-grid slds-gutters"> <div class="slds-col"> <lightning-record-view-form record-id={recordId} object-api-name={objectApiName}> <div class="slds-grid slds-gutters"> <div class="slds-col slds-size_1-of-2"> <lightning-output-field field-name="Name"></lightning-output-field> </div> <!-- Add additional fields as needed --> </div> </lightning-record-view-form> </div> </div> </template> <template if:true={error}> <p>Error loading record data</p> </template> </div> </lightning-card> </template>
ldsExample.js
:
// ldsExample.js import { LightningElement, api, wire } from 'lwc'; import getRecordId from '@salesforce/apex/ExampleController.getRecordId'; export default class LdsExample extends LightningElement { @api recordId; objectApiName = 'Account'; // Change to the desired object API name @wire(getRecordId, { recordId: '$recordId' }) wiredRecord({ error, data }) { if (data) { // Handle the record data console.log('Record data:', data); this.record = data; this.error = undefined; } else if (error) { // Handle the error console.error('Error loading record:', error); this.error = error; this.record = undefined; } } // Additional logic can be added as needed }
ldsExample.js-meta.xml
:
<!-- ldsExample.js-meta.xml --> <?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="ldsExample"> <apiVersion>52.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__AppPage</target> <target>lightning__RecordPage</target> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
- Wire Up the Component: Use the
ldsExample
component in your Lightning App Builder, Record Page, or Home Page. Ensure that theobjectApiName
property is set to the desired Salesforce object API name. - Test the Component: When you place the
ldsExample
component on a record page, it will automatically fetch and display details about the record. Thelightning-record-view-form
component, combined with Lightning Data Service, simplifies the process of displaying record details in Lightning Web Components.
Remember to adjust the objectApiName
property in the component based on the Salesforce object you want to display. Additionally, you can customize the Lightning card and add more fields as needed.
- Implement Caching:
- Implement client-side caching to store fetched data temporarily and avoid unnecessary server calls.
- Be cautious with caching stale data; implement mechanisms to refresh the cache when needed.
In Salesforce Lightning Web Components (LWC), there isn’t a built-in caching mechanism for data. However, you can implement your own caching strategy using JavaScript to store and retrieve data. In the example below, we’ll use the browser’s localStorage
to cache data. Keep in mind that localStorage
has limitations, such as a storage capacity of about 5 MB and being synchronous, which might affect performance.
Here’s a basic example of implementing caching in an LWC:
- Create a caching service (
cacheService.js
):
// cacheService.js const CACHE_PREFIX = 'myAppCache_'; export const setCache = (key, data) => { try { localStorage.setItem(CACHE_PREFIX + key, JSON.stringify(data)); } catch (error) { console.error('Error setting cache:', error); } }; export const getCache = (key) => { try { const cachedData = localStorage.getItem(CACHE_PREFIX + key); return cachedData ? JSON.parse(cachedData) : null; } catch (error) { console.error('Error getting cache:', error); return null; } };
- Use the caching service in your LWC (
cachedComponent.js
):
// cachedComponent.js import { LightningElement, track } from 'lwc'; import { setCache, getCache } from 'c/cacheService'; export default class CachedComponent extends LightningElement { @track data; connectedCallback() { // Try to retrieve data from the cache this.data = getCache('myData'); // If data is not in the cache, fetch it from the server if (!this.data) { this.fetchDataFromServer(); } } fetchDataFromServer() { // Simulating a server request // In a real-world scenario, you would use an Apex method or call an external API setTimeout(() => { const newData = { message: 'Data fetched from the server' }; this.data = newData; // Cache the data for future use setCache('myData', newData); }, 2000); // Simulating a 2-second delay } }
- Wire up the LWC (
cachedComponent.html
):
<!-- cachedComponent.html --> <template> <div> <template if:true={data}> <p>{data.message}</p> </template> <template if:false={data}> <p>Loading...</p> </template> </div> </template>
- Metadata file for the LWC (
cachedComponent.js-meta.xml
):
<!-- cachedComponent.js-meta.xml --> <?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="cachedComponent"> <apiVersion>52.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__AppPage</target> <target>lightning__RecordPage</target> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
In this example, the cacheService.js
file provides two functions, setCache
and getCache
, to store and retrieve data from localStorage
. The cachedComponent.js
LWC component uses these functions to check for cached data when it’s loaded. If the data is not found in the cache, it fetches the data from the server and stores it in the cache for future use.
Please note that caching strategies can vary based on your specific use case and requirements. Consider the limitations of localStorage
and explore other caching options if needed, such as using IndexedDB or a service worker for more advanced scenarios.
- Throttling and Debouncing:
- Implement throttling and debouncing for user input actions that trigger data fetching.
- Throttling prevents the execution of a function more than once in a specified time period, while debouncing delays the execution of a function until after a certain amount of time has passed since the last invocation.
Throttling and debouncing are techniques used to control the rate at which a function is called. Throttling ensures that a function is called at a steady rate, while debouncing delays the execution of a function until a certain amount of time has passed since the last invocation. These techniques are often used in scenarios like handling user input, resizing events, or other situations where frequent calls to a function may be resource-intensive.
Here’s an example of implementing throttling and debouncing in a Lightning Web Component (LWC) using JavaScript:
- Create the Throttling Service (
throttleService.js
):
// throttleService.js export const throttle = (func, limit) => { let lastFunc; let lastRan; return function () { const context = this; const args = arguments; if (!lastRan) { func.apply(context, args); lastRan = Date.now(); } else { clearTimeout(lastFunc); lastFunc = setTimeout(function () { if ((Date.now() - lastRan) >= limit) { func.apply(context, args); lastRan = Date.now(); } }, limit - (Date.now() - lastRan)); } }; };
- Create the Debouncing Service (
debounceService.js
):
// debounceService.js export const debounce = (func, delay) => { let timeoutId; return function () { const context = this; const args = arguments; clearTimeout(timeoutId); timeoutId = setTimeout(() => { func.apply(context, args); }, delay); }; };
- Use Throttling and Debouncing in Your LWC (
throttleDebounceExample.js
):
// throttleDebounceExample.js import { LightningElement } from 'lwc'; import { throttle } from 'c/throttleService'; import { debounce } from 'c/debounceService'; export default class ThrottleDebounceExample extends LightningElement { handleThrottledInput = throttle(this.handleInput, 1000); // Throttle every 1000ms (1 second) handleDebouncedInput = debounce(this.handleInput, 500); // Debounce with a delay of 500ms handleInput(event) { const inputValue = event.target.value; console.log('Input Value:', inputValue); // Perform your logic here based on the input value } handleThrottledInputChange(event) { this.handleThrottledInput(event); } handleDebouncedInputChange(event) { this.handleDebouncedInput(event); } }
- Wire up the LWC (
throttleDebounceExample.html
):
<!-- throttleDebounceExample.html --> <template> <div> <label>Throttled Input:</label> <lightning-input oninput={handleThrottledInputChange}></lightning-input> <label>Debounced Input:</label> <lightning-input oninput={handleDebouncedInputChange}></lightning-input> </div> </template>
- Metadata file for the LWC (
throttleDebounceExample.js-meta.xml
):
<!-- throttleDebounceExample.js-meta.xml --> <?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="throttleDebounceExample"> <apiVersion>52.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__AppPage</target> <target>lightning__RecordPage</target> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
In this example, the throttleService.js
and debounceService.js
files provide the throttle
and debounce
functions, respectively. The throttleDebounceExample.js
LWC component uses these functions to handle throttled and debounced input events. Adjust the time intervals (e.g., 1000
milliseconds for throttling and 500
milliseconds for debouncing) based on your specific requirements.
This component includes two input fields: one for throttled input and one for debounced input. The throttling and debouncing functions ensure that the handleInput
function is called at a controlled rate, avoiding excessive calls in response to rapid user input.
- Optimized Queries:
- Optimize your Apex queries to retrieve only the necessary fields and filter records efficiently.
- Leverage query optimizations such as indexing on fields frequently used in filters.
- Use Lightning Datatable Features:
- Utilize the built-in features of the Lightning Datatable component, such as pagination and dynamic loading.
- Implement custom logic to control the number of rows fetched at a time.
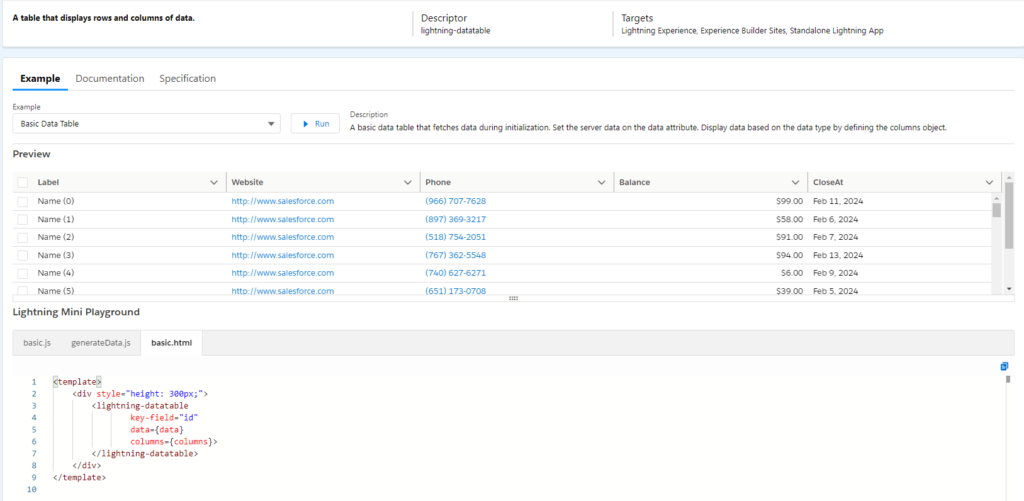
- Progressive Loading:
- Implement progressive loading to gradually render data as it becomes available.
- This can be achieved by loading a small initial dataset and then fetching additional data as the user interacts with the component.
Progressive loading, also known as infinite scrolling, is a technique where additional content is loaded as the user scrolls down a page. This is commonly used to improve the user experience by fetching and rendering data on demand, rather than loading everything upfront. In Lightning Web Components (LWC), you can implement progressive loading by handling scroll events and fetching additional data when needed.
Here’s a simple example of progressive loading in LWC:
- Create the Progressive Loading Component (
progressiveLoadingExample.js
):
// progressiveLoadingExample.js import { LightningElement, track } from 'lwc'; export default class ProgressiveLoadingExample extends LightningElement { @track items = []; @track isLoading = false; connectedCallback() { // Load initial set of data this.loadMoreData(); } loadMoreData() { // Simulating an asynchronous data fetch this.isLoading = true; setTimeout(() => { const newData = this.generateData(); this.items = [...this.items, ...newData]; this.isLoading = false; }, 1000); // Simulating a 1-second delay } generateData() { // Generate sample data for illustration const newData = []; for (let i = 0; i < 10; i++) { newData.push(`Item ${this.items.length + i + 1}`); } return newData; } handleScroll(event) { // Load more data when user scrolls to the bottom const { scrollTop, scrollHeight, clientHeight } = event.target; if (scrollTop + clientHeight >= scrollHeight - 100 && !this.isLoading) { this.loadMoreData(); } } }
- Wire Up the Component (
progressiveLoadingExample.html
):
<!-- progressiveLoadingExample.html --> <template> <div onscroll={handleScroll} style="height: 300px; overflow-y: auto; border: 1px solid #ccc;"> <template for:each={items} for:item="item"> <div key={item} class="slds-p-around_medium">{item}</div> </template> <template if:true={isLoading}> <div class="slds-p-around_medium">Loading...</div> </template> </div> </template>
- Metadata file for the Component (
progressiveLoadingExample.js-meta.xml
):
<!-- progressiveLoadingExample.js-meta.xml --> <?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="progressiveLoadingExample"> <apiVersion>52.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__AppPage</target> <target>lightning__RecordPage</target> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
- Style the Component (
progressiveLoadingExample.css
):
/* progressiveLoadingExample.css */ .slds-p-around_medium { border-bottom: 1px solid #ccc; }
In this example, the ProgressiveLoadingExample
component loads an initial set of data in the connectedCallback
lifecycle hook. As the user scrolls down, the handleScroll
method is triggered, and if the scroll position is near the bottom (within 100 pixels), additional data is loaded asynchronously using the loadMoreData
method.
The isLoading
property is used to display a loading message while data is being fetched. The generateData
method is used to simulate the creation of new data items. Adjust the logic and styles based on your specific requirements.
Remember to adjust the styles and content to fit the design and structure of your application.
- Error Handling:
- Implement robust error handling to gracefully manage situations where data retrieval fails.
- Provide meaningful error messages to users and log errors for developers.
- Testing and Optimization:
- Test the performance of your component with large datasets to identify bottlenecks.
- Optimize your component code and use browser developer tools to identify performance issues.