Introduction:
Data security is vital in any application to protect sensitive information. In Salesforce development, we often deal with sensitive data, such as customer details or financial information. While Salesforce offers built-in security features, sometimes we need extra protection for our data. In this blog post, we’ll learn how to add a layer of security by Data Encryption and Decryption in Lightning Web Components, Salesforce’s modern UI framework.
Understanding Encryption and Decryption:
Encryption is like putting your data in a secret box. You use a key to lock the box (encrypt) so that only those with the right key can open it (decrypt) and see what’s inside. This helps keep your data safe from prying eyes, especially when it’s being sent over the internet or stored on servers.
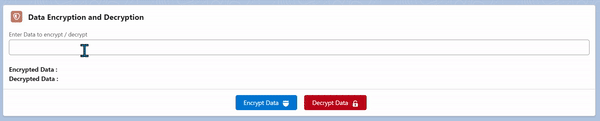
Implementing Custom Data Encryption in LWC:
To encrypt data in LWC, we’ll use a library called CryptoJS. Think of it as a toolkit for locking and unlocking data. Here’s a simple example of how to encrypt & decrypt data using CryptoJS:
<!-- @description : @author : Abhishek Verma @group : @last modified on : 02-14-2024 @last modified by : Abhishek Verma --> <template> <lightning-card variant="Narrow" title="Data Encryption and Decryption" icon-name="custom:custom91"> <div class="slds-p-horizontal_small"> <lightning-input type="text" label="Enter Data to encrypt / decrypt " value={Orgplaintext} onchange={handleChanges}></lightning-input> </div> <br> <div class="slds-p-horizontal_small"> <p> <b>Encrypted Data : </b>{encryptedData}</p> <p><b>Decrypted Data : </b>{decryptedData}</p> </div> <p slot="footer"> <lightning-button variant="brand" label="Encrypt Data" icon-name="utility:shield" icon-position="right" onclick={handleEncrypt} class="slds-m-left_x-small"></lightning-button> <lightning-button variant="destructive" label="Decrypt Data" onclick={handleDecrypt} icon-name="utility:unlock" class="slds-m-left_x-small" icon-position="right"></lightning-button> </p> </lightning-card> </template>
import { LightningElement } from 'lwc'; import { loadScript } from 'lightning/platformResourceLoader'; import cryptoJS from '@salesforce/resourceUrl/cryptoJS'; export default class EncryptionComponent extends LightningElement { encryptedData; decryptedData; Orgplaintext; connectedCallback() { // Load CryptoJS script once the component is rendered Promise.all([ loadScript(this, cryptoJS) ]).then(() => { // CryptoJS script loaded successfully console.log('CryptoJS loaded'); }).catch(error => { // Error loading CryptoJS script console.error('Error loading CryptoJS:', error); }); } handleChanges(event){ this.Orgplaintext = event.target.value; } handleEncrypt() { console.log('this.Orgplaintext',this.Orgplaintext); const plaintext = this.Orgplaintext;//'Sensitive data to be encrypted'; const encryptionKey = 'SecretEncryptionKey'; const ciphertext = CryptoJS.AES.encrypt(plaintext, encryptionKey).toString(); this.encryptedData = ciphertext; console.log('Encrypted Data:', ciphertext); } handleDecrypt() { const ciphertext = this.encryptedData; // Encrypted data const decryptionKey = 'SecretEncryptionKey'; console.log('this.Orgplaintext',this.encryptedData); const bytes = CryptoJS.AES.decrypt(ciphertext, decryptionKey); const plaintext = bytes.toString(CryptoJS.enc.Utf8); console.log('Decrypted Data:', plaintext); this.decryptedData = plaintext; } }
In this code, we take some sensitive data (Orgplaintext), like a password or credit card number, and a secret key (encryptionKey). Then, we use CryptoJS to encrypt & decrypt the data using the AES algorithm.
Conclusion:
By using custom data encryption and decryption in Lightning Web Components, we can add an extra layer of security to our applications. This helps protect sensitive information from unauthorized access. Just remember to keep your encryption keys safe and follow best practices for security. With proper implementation, we can enhance the overall security of our Salesforce applications and keep our users’ data safe.
Resource: crypto-js.min.js