The blog post “Salesforce Integration Interview Questions Answers” is your ultimate guide for preparing for Salesforce Integration interviews. This guide is useful whether you’re an experienced Salesforce developer aiming to broaden your abilities or a job applicant looking to enter the Salesforce Integration domain. It includes a comprehensive selection of questions, which range from basic concepts to more complex strategies of integration.
1. What is Salesforce Integration, and why is it important?
Answer: Salesforce Integration involves connecting Salesforce with other applications or systems to exchange data seamlessly. It is crucial for businesses to have a unified view of their data across various platforms.
2. Explain the different types of Salesforce Integration.
Answer: There are several types of Salesforce Integration, including:
- HTTP Callouts: Invoking external web services.
- SOAP Integration: Using Simple Object Access Protocol for communication.
- REST Integration: Using Representational State Transfer for web services.
- Bulk API: Mass data transfers for large datasets.
- Streaming API: Real-time data synchronization.
3. How can you make an HTTP Callout in Apex?
Answer: You can make an HTTP Callout in Apex using the HttpRequest
and HttpResponse
classes. Here’s a simple example:
Http http = new Http(); HttpRequest request = new HttpRequest(); request.setEndpoint('https://example.com/api'); request.setMethod('GET'); HttpResponse response = http.send(request); System.debug('Response Body: ' + response.getBody());
4. What is the difference between REST and SOAP integration in Salesforce?
Answer: REST (Representational State Transfer) is a lightweight, stateless protocol, while SOAP (Simple Object Access Protocol) is a heavyweight, stateful protocol. REST is simpler, uses standard HTTP methods, and is generally faster, whereas SOAP is more standardized, supports transactions, and has built-in security features.
5. How do you handle errors in Salesforce Integration?
Answer: You can handle errors in Salesforce Integration by checking the response status code and handling exceptions in your Apex code. For example:
HttpResponse response = http.send(request); if (response.getStatusCode() != 200) { // Handle error, log or throw an exception }
6. Explain the use case for the Bulk API in Salesforce Integration.
Answer: The Bulk API is used for mass data transfers when dealing with large datasets. It’s efficient for loading or deleting a large volume of records asynchronously.
7. How can you implement a RESTful web service in Salesforce?
Answer: You can implement a RESTful web service in Salesforce using the @RestResource
annotation. Here’s a simple example:
@RestResource(urlMapping='/exampleEndpoint/*') global with sharing class MyRestResource { @HttpGet global static String doGet() { return 'Hello, World!'; } }
8. What is an Outbound Message in Salesforce Integration?
Answer: An Outbound Message is a part of Workflow Rule that sends information to an external service in the form of SOAP messages when the rule criteria are met.
9. How do you handle governor limits in Salesforce Integration?
Answer: Governor limits in Salesforce, such as SOQL queries and DML operations, must be considered. Bulkifying your code, using asynchronous processing, and optimizing queries are common strategies.
10. Explain the use case for Streaming API in Salesforce Integration.
Answer: Streaming API is used for real-time data synchronization. It allows Salesforce to push changes to external systems immediately as they occur, ensuring up-to-date information.
his example demonstrates a simple use case where updates to the “Account” object trigger real-time notifications to the UI using the Streaming API.
Apex Class (StreamingAPIExampleController.cls):
public class StreamingAPIExampleController { // This method will be called whenever an Account record is updated public void handleAccountUpdate(List<Account> newAccounts, Map<Id, Account> oldAccountMap) { // Prepare the data you want to send in the notification List<Map<String, Object>> accountUpdates = new List<Map<String, Object>>(); for (Account newAccount : newAccounts) { Map<String, Object> updateData = new Map<String, Object>(); updateData.put('AccountId', newAccount.Id); updateData.put('AccountName', newAccount.Name); // Add more fields as needed accountUpdates.add(updateData); } // Publish the notification to the subscribed clients StreamingAPIHelper.publishUpdate('/topic/AccountUpdates', accountUpdates); } }
Apex Class (StreamingAPIHelper.cls):
public class StreamingAPIHelper { // Helper method to publish updates to a specific topic public static void publishUpdate(String channel, Object payload) { String jsonString = JSON.serialize(payload); StreamingAPI.PublishResult[] publishResults = StreamingAPI.publish(channel, jsonString); // Check publish results if needed } }
Visualforce Page (StreamingAPIExamplePage.page):
<apex:page controller="StreamingAPIExampleController"> <apex:includeScript value="/cometd/cometd.js" /> <apex:includeScript value="/cometd/jquery.cometd.js" /> <script> $(document).ready(function() { // Connect to the CometD endpoint $.cometd.configure({ url: window.location.protocol + '//' + window.location.host + '/cometd/42.0/', requestHeaders: { Authorization: 'OAuth {!$Api.Session_Id}' } // Use the Salesforce session ID }); $.cometd.handshake(); // Subscribe to the AccountUpdates channel $.cometd.subscribe('/topic/AccountUpdates', function(message) { // Handle incoming messages (notifications about Account updates) console.log('Received message:', message); // Update your UI or perform other actions based on the incoming data }); }); </script> </apex:page>
11. How can you handle authentication in Salesforce Integration?
Answer: Salesforce supports various authentication mechanisms such as OAuth, Username-Password Flow, and JWT (JSON Web Token). The choice depends on the integration scenario and security requirements. For example, using OAuth 2.0 for external system authentication:
HttpRequest request = new HttpRequest(); request.setEndpoint('https://login.salesforce.com/services/oauth2/token'); request.setMethod('POST'); request.setHeader('Content-Type', 'application/x-www-form-urlencoded'); request.setBody('grant_type=password&client_id=yourClientId&client_secret=yourClientSecret&username=yourUsername&password=yourPassword'); Http http = new Http(); HttpResponse response = http.send(request); String accessToken = JSON.deserialize(response.getBody(), Map<String, String>.class).get('access_token');
12. Explain the considerations for handling large data volumes in Salesforce Integration.
Answer: Large data volumes require careful consideration to avoid hitting governor limits. Implementing bulkified operations, using Bulk API for data transfers, and optimizing queries are essential. Pagination techniques should be employed when dealing with large result sets.
13. What is the use of the Metadata API in Salesforce Integration?
Answer: The Metadata API allows developers to retrieve, deploy, create, update, or delete customizations for Salesforce organizations. It is commonly used for managing custom metadata, profiles, and settings.
Below is a simple example of how you might use the Metadata API in Salesforce using Apex
Apex Class (MetadataAPIExample.cls):
public class MetadataAPIExample { // Method to create a custom object using Metadata API public static void createCustomObject(String objectName) { MetadataService.MetadataPort service = createMetadataService(); // Create CustomObject metadata MetadataService.CustomObject customObject = new MetadataService.CustomObject(); customObject.fullName = objectName + '__c'; customObject.label = objectName; customObject.pluralLabel = objectName + 's'; customObject.nameField = new MetadataService.CustomField(); customObject.nameField.type_x = 'Text'; customObject.nameField.label = 'Custom ' + objectName + ' Name'; // Create CustomField metadata MetadataService.CustomField customField = new MetadataService.CustomField(); customField.fullName = objectName + '__c.CustomField__c'; customField.label = 'Custom Field'; customField.type_x = 'Text'; // Add the custom field to the custom object customObject.fields = new MetadataService.CustomField[] { customField }; // Create the custom object List<MetadataService.SaveResult> results = service.createMetadata(new MetadataService.Metadata[] { customObject }); // Check for success or failure for (MetadataService.SaveResult result : results) { if (result.success) { System.debug(objectName + ' object created successfully!'); } else { System.debug('Error creating ' + objectName + ' object: ' + result.errors[0].message); } } } // Helper method to create and configure the MetadataService private static MetadataService.MetadataPort createMetadataService() { MetadataService.MetadataPort service = new MetadataService.MetadataPort(); service.SessionHeader = new MetadataService.SessionHeader_element(); service.SessionHeader.sessionId = UserInfo.getSessionId(); return service; } }
This example includes a method createCustomObject
that creates a custom object with a custom field using the Metadata API. It utilizes the MetadataService
class, which represents the Metadata API in Salesforce.
14. How can you implement a webhook in Salesforce?
Answer: Implementing a webhook in Salesforce involves creating an Apex REST class annotated with @RestResource
and handling the incoming HTTP request. Here’s a basic example:
@RestResource(urlMapping='/webhookEndpoint/*') global with sharing class MyWebhookResource { @HttpPost global static String doPost(String requestBody) { // Process incoming webhook data return 'Webhook processed successfully'; } }
15. Explain the use of Named Credentials in Salesforce Integration.
Answer: Named Credentials securely store authentication details and can be used in Apex code or the declarative setup for authenticating to external services. They abstract sensitive information, providing a more secure and manageable way to handle authentication.
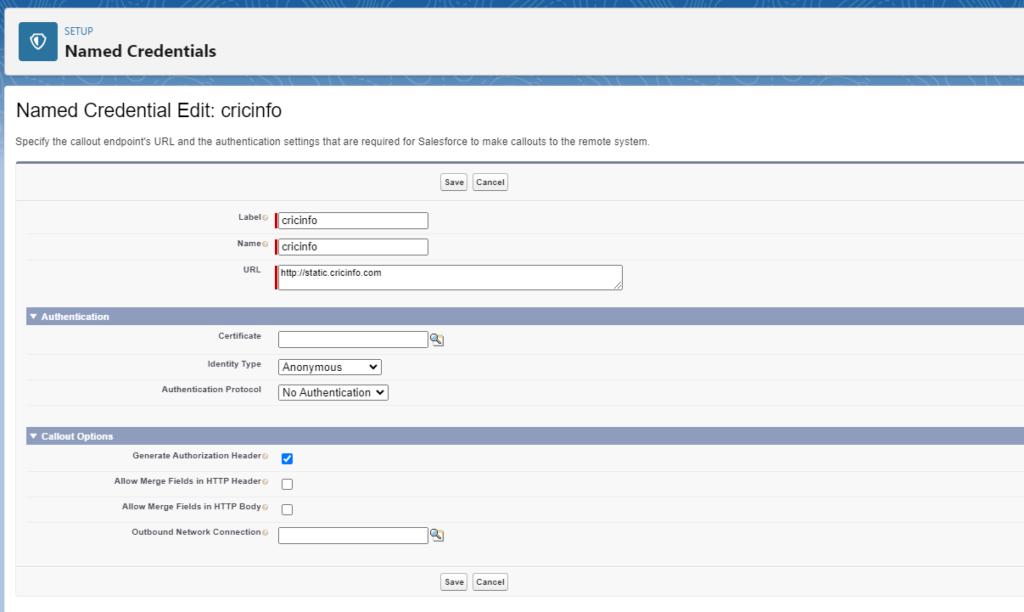
16. How do you implement a two-way integration between Salesforce and an external system?
Answer: Two-way integration involves sending data from Salesforce to an external system and vice versa. This can be achieved using a combination of Flow, Apex callouts, platform events, and middleware solutions like MuleSoft or Boomi.
17. What is the significance of the Composite API in Salesforce Integration?
Answer: The Composite API allows you to make multiple requests in a single call, reducing the number of round trips to the server. It is beneficial for improving performance and managing complex transactions.
18. How can you schedule data integration jobs in Salesforce?
Answer: You can use Salesforce’s built-in scheduling features like Scheduled Jobs (Apex Scheduler) or leverage external tools such as Heroku Scheduler to trigger integration jobs at predefined intervals.
19. Explain the considerations for integrating Salesforce with external databases.
Answer: When integrating with external databases, considerations include data mapping, ensuring data consistency, handling bulk data transfers efficiently, and maintaining proper security measures. Connection pooling and optimizing queries are crucial for performance.
20. Describe the use case for Platform Events in Salesforce Integration.
Answer: Platform Events enable event-driven architecture in Salesforce. They can be used to broadcast changes within Salesforce or trigger external processes, allowing systems to react in real-time to data changes.
21. How can you implement a callout retry mechanism in Salesforce?
Answer: Implementing a callout retry mechanism involves catching exceptions, checking for specific HTTP status codes, and retrying the call after a delay. Here’s a basic example:
Integer maxAttempts = 3; Integer currentAttempt = 0; do { try { // Make the HTTP callout // ... break; // Break the loop if successful } catch (Exception ex) { // Log the exception or take appropriate action currentAttempt++; if (currentAttempt < maxAttempts) { // Wait for a delay before retrying Thread.sleep(5000); // 5 seconds delay (adjust as needed) } else { throw ex; // Throw the exception if max attempts reached } } } while (currentAttempt < maxAttempts);
22. Explain the use case for the Tooling API in Salesforce Integration.
Answer: The Tooling API is used for building custom development tools or integrating Salesforce with external development environments. It provides metadata information and enables developers to interact with their Salesforce org programmatically.
Here’s a simple example of using the Tooling API in Salesforce, specifically querying Apex classes.
Apex Class (ToolingAPIExample.cls):
public class ToolingAPIExample { // Method to query Apex classes using Tooling API public static void queryApexClasses() { String query = 'SELECT Id, Name, Body FROM ApexClass'; // Make a callout to Tooling API HttpRequest request = createHttpRequest('GET', '/services/data/v52.0/tooling/query?q=' + EncodingUtil.urlEncode(query, 'UTF-8')); HttpResponse response = sendHttpRequest(request); // Parse and handle the response if (response.getStatusCode() == 200) { // Successful response Map<String, Object> jsonResponse = (Map<String, Object>)JSON.deserializeUntyped(response.getBody()); List<Object> records = (List<Object>)jsonResponse.get('records'); for (Object record : records) { Map<String, Object> recordMap = (Map<String, Object>)record; System.debug('Apex Class Name: ' + recordMap.get('Name')); System.debug('Apex Class Body: ' + recordMap.get('Body')); } } else { // Error handling System.debug('Error querying Apex classes: ' + response.getBody()); } } // Helper method to create HTTP request private static HttpRequest createHttpRequest(String method, String endpoint) { HttpRequest request = new HttpRequest(); request.setEndpoint(URL.getSalesforceBaseUrl().toExternalForm() + endpoint); request.setMethod(method); request.setHeader('Authorization', 'Bearer ' + UserInfo.getSessionId()); return request; } // Helper method to send HTTP request private static HttpResponse sendHttpRequest(HttpRequest request) { Http http = new Http(); return http.send(request); } }
This example includes a method queryApexClasses
that queries Apex classes using the Tooling API. It makes a callout to the Tooling API’s query
endpoint to retrieve information about Apex classes.
Usage:
You can then use the ToolingAPIExample
class to query Apex classes by calling the queryApexClasses
method:
ToolingAPIExample.queryApexClasses();
Ensure that your Salesforce user has the necessary permissions to access the Tooling API. Also, note that callouts from Apex classes are subject to various limits and considerations, such as governor limits and authentication requirements.
23. How can you handle bulk data deletion efficiently in Salesforce Integration?
Answer: Use the Bulk API for mass data deletion to optimize performance. The Bulk API supports the delete
operation for large volumes of records. Additionally, consider using batch processing to break down the deletion into smaller chunks.
24. What is the difference between Outbound Messaging and Apex Callouts in Salesforce Integration?
Answer: Outbound Messaging is a declarative approach that involves configuring workflow rules to send SOAP messages to external services. Apex Callouts, on the other hand, are programmatically implemented using Apex code and support both SOAP and REST protocols.
25. Explain the use of Named Credentials in relation to Callouts.
Answer: Named Credentials can be used in Apex code for callouts to external services. They securely store authentication information, eliminating the need to hardcode sensitive data in your code.
26. How can you handle schema changes in the external system affecting Salesforce Integration?
Answer: To handle schema changes, use dynamic Apex to adapt to changes in the external system’s structure. Techniques such as JSON deserialization or dynamic SOQL can be employed to work with flexible data structures.
27. What is the use case for Change Data Capture (CDC) in Salesforce Integration?
Answer: Change Data Capture allows you to identify and capture changes in Salesforce records, providing a reliable way to integrate with external systems by sending notifications about data changes, thus eliminating the need for frequent polling.
28. How do you handle session management in long-running integrations?
Answer: For long-running integrations, consider using the asynchronous processing capabilities of Salesforce, such as future methods or batch jobs. These allow you to handle large data volumes and avoid hitting processing time limits.
29. Explain the considerations for integrating Salesforce with on-premises systems.
Answer: Integrating with on-premises systems often involves using middleware solutions like MuleSoft or Boomi to bridge the gap between cloud and on-premises environments. Security measures, such as VPNs or secure gateways, should be considered.
30. Describe the role of External Services in Salesforce Integration.
Answer: External Services provide a declarative way to integrate with external systems. By defining an external service, you can generate Apex code that acts as a proxy for the external service, simplifying integration implementation.
Certainly! Here are 10 more Salesforce Integration interview questions along with their answers:
31. How do you handle asynchronous processing in Salesforce Integration?
Answer: Asynchronous processing in Salesforce can be achieved using tools like Apex Batch, Queueable Jobs, or future methods. These allow you to offload processing to the background, avoiding synchronous limits.
32. Explain the use case for Named Credentials with OAuth 2.0 Authentication.
Answer: Named Credentials simplify OAuth 2.0 authentication by securely storing the authentication details. When configuring a Named Credential with OAuth, Salesforce handles the token acquisition and renewal, making it easier to integrate with services requiring OAuth.
33. What is the External Objects feature in Salesforce, and how does it relate to integration?
Answer: External Objects represent data stored outside Salesforce, typically in an external database. They enable real-time access to external data, allowing you to integrate and interact with external databases seamlessly.
34. How can you implement a real-time integration using Platform Events?
Answer: Platform Events enable real-time integration by broadcasting events when certain conditions are met. Subscribers, which can be external systems, listen for these events and take action in real-time.
35. Explain the concept of Apex Triggers in Salesforce Integration.
Answer: Apex Triggers are pieces of code that execute before or after specific events occur in Salesforce, such as record creation, update, or deletion. Triggers can be used to initiate integration processes based on changes in Salesforce data.
36. What is the Remote Site Settings in Salesforce, and why is it important for integrations?
Answer: Remote Site Settings allow Salesforce to make HTTP requests to external services. When integrating with an external service, you need to add the service’s endpoint to the Remote Site Settings to whitelist it.
37. How can you ensure data consistency in a bi-directional Salesforce Integration?
Answer: Data consistency can be maintained by implementing proper error handling, transaction management, and reconciliation mechanisms. Using a middleware solution or leveraging Idempotent APIs can also contribute to ensuring consistency.
38. Explain the concept of Composite Resources in the Composite API.
Answer: Composite Resources in the Composite API allow you to group multiple subrequests into a single composite request, reducing the number of round trips and optimizing performance.
39. What is the Bulk Query API, and how can it be useful in Salesforce Integration?
Answer: The Bulk Query API allows you to query large sets of data efficiently. It’s beneficial when dealing with complex queries or when you need to retrieve a large amount of data from Salesforce.
40. How can you monitor and troubleshoot integration issues in Salesforce?
Answer: Monitoring and troubleshooting integration issues involve utilizing Salesforce’s debug logs, system logs, and monitoring tools. Additionally, external tools like Postman or cURL can be used to test and diagnose issues with external services.
41. Explain the considerations for integrating Salesforce with IoT (Internet of Things) devices.
Answer: Integrating with IoT devices may involve using platforms like Heroku for real-time data processing, implementing Streaming API for event-driven architecture, and ensuring secure communication with devices through protocols like MQTT or HTTP.
Certainly! Here are additional Salesforce Integration interview questions and answers:
42. What is the use of External Services in Salesforce, and how does it simplify integration?
Answer: External Services provide a declarative way to define and consume external services. By importing an OpenAPI (formerly known as Swagger) specification, you can generate Apex classes that act as a proxy for the external service, simplifying integration implementation.
43. Explain the considerations for integrating Salesforce with a legacy SOAP-based system.
Answer: When integrating with a legacy SOAP-based system, understanding the WSDL (Web Services Description Language) is crucial. Use the WSDL to generate Apex classes and handle SOAP headers and authentication appropriately.
44. How do you implement pagination in Salesforce Integration when dealing with large datasets?
Answer: Pagination is crucial for handling large datasets. When making queries to an external service or Salesforce, use limit and offset parameters or implement cursor-based pagination to retrieve data in smaller, manageable chunks.
45. Describe the role of Middleware in Salesforce Integration and provide examples of popular middleware solutions.
Answer: Middleware acts as an intermediary layer between different applications, facilitating communication and data exchange. Examples of popular middleware solutions for Salesforce Integration include MuleSoft, Dell Boomi, and Apache Camel.
46. What is the difference between the Streaming API and Platform Events in terms of real-time integration?
Answer: While both Streaming API and Platform Events enable real-time integration, the Streaming API is generally used for pushing changes within Salesforce, while Platform Events provide a more generalized and scalable event-driven architecture for both internal and external systems.
47. How can you handle composite requests in RESTful integration using the Composite API in Salesforce?
Answer: Composite requests allow you to group multiple subrequests into a single request, improving efficiency. When using the Composite API, you can structure your requests in a composite resource to execute multiple operations in a single call.
48. Explain the considerations for integrating Salesforce with an ERP (Enterprise Resource Planning) system.
Answer: Integrating with an ERP system requires careful data mapping, handling of transactional data, and understanding the business processes. Consider using middleware to synchronize data between Salesforce and the ERP system in real-time or through scheduled batches.
49. What is the purpose of the Connected App in Salesforce, and how is it relevant to integrations?
Answer: A Connected App in Salesforce represents an external application that integrates with Salesforce. It is essential for securely authenticating and authorizing external applications to access Salesforce data via APIs.
50. How can you secure data transmission in Salesforce Integration, especially when dealing with sensitive information?
Answer: To secure data transmission, use HTTPS (SSL/TLS) for encrypted communication. Additionally, consider using OAuth for authentication and authorization, and ensure that sensitive information such as credentials is stored securely using techniques like Named Credentials.
51. Explain the role of Change Data Capture (CDC) in a data synchronization scenario.
Answer: Change Data Capture is used for tracking changes to Salesforce records. In a data synchronization scenario, CDC enables you to capture and propagate only the changes that have occurred since the last synchronization, reducing the amount of data transferred.
Subscribe to my YouTube channel for more exciting videos on salesforce 👇