In the world of Salesforce, delivering a seamless user experience is key to driving engagement and success. Custom Navigation in Lightning Web Components (LWC) plays a pivotal role in achieving this by allowing developers to create dynamic, user-friendly interfaces. Whether you’re building a complex enterprise-grade application or a simple project, mastering Custom Navigation combined with the NavigationMixin can significantly elevate your application’s interactivity and user experience.
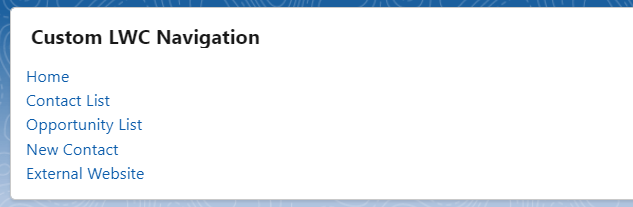
Understanding Custom Navigation in Lightning Web Components
What is Custom Navigation?
At its core, Custom Navigation in Lightning Web Components is about creating navigation patterns that are tailored to your application’s specific needs, beyond the standard navigation capabilities provided by Salesforce. This customizability ensures that users can move through your application efficiently, accessing the information they need without unnecessary clicks or confusion.
Why is Custom Navigation Important?
Custom Navigation is crucial for several reasons. It enhances the user experience by making applications more intuitive and easier to use, which in turn can increase user adoption rates. Additionally, it allows developers to build highly interactive and user-centric apps that stand out in the crowded Salesforce ecosystem.
The Power of NavigationMixin
NavigationMixin is a powerful tool that, when used correctly, significantly simplifies the development of custom navigation in LWC. It provides developers with a set of methods that can be used to programmatically navigate to Salesforce pages, including object pages, record detail pages, and custom pages, among others. This power and flexibility are invaluable when creating dynamic, user-focused applications.
Step-by-Step Guide to Implementing Custom Navigation
Preparing Your Environment
Before diving into coding, ensure your Salesforce environment is set up correctly, including the necessary permissions and settings to create Lightning Web Components.
Creating Your First Custom Navigation Component
- Start by creating a new LWC in your VS Code.
- Integrate the NavigationMixin to incorporate custom navigation functionality into your component.
Implementing Custom Navigation Menu
In your CustomNavigationMenu.html
file, define your navigation menu:
<!-- @description : @author : Abhishek Verma @group : @last modified on : 02-14-2024 @last modified by : Abhishek Verma --> <template> <lightning-card variant="Narrow" title="Custom LWC Navigation" > <div class="slds-p-horizontal_small"> <!-- Card Body --> <div class="navigation-menu"> <ul> <li><a href="#" onclick={navigateToHome}>Home</a></li> <li><a href="#" onclick={navigateToContactList}>Contact List</a></li> <li><a href="#" onclick={navigateToOpportunityList}>Opportunity List</a></li> <li><a href="#" onclick={navigateToNewContact}>New Contact</a></li> <li><a href="#" onclick={navigateToExternal}>External Website</a></li> </ul> </div> </div> </lightning-card> </template>
Implementing Navigation Logic
In the JavaScript file (CustomNavigationMenu.js
), implement the navigation logic using NavigationMixin
:
import { LightningElement, wire } from 'lwc'; import { NavigationMixin } from 'lightning/navigation'; export default class CustomNavigationMenu extends NavigationMixin(LightningElement) { navigateToHome() { this[NavigationMixin.Navigate]({ type: 'standard__namedPage', attributes: { pageName: 'home' } }); } navigateToContactList() { this[NavigationMixin.Navigate]({ type: 'standard__objectPage', attributes: { objectApiName: 'Contact', actionName: 'list' } }); } navigateToOpportunityList() { this[NavigationMixin.Navigate]({ type: 'standard__objectPage', attributes: { objectApiName: 'Opportunity', actionName: 'list' } }); } navigateToNewContact() { this[NavigationMixin.Navigate]({ type: 'standard__objectPage', attributes: { objectApiName: 'Contact', actionName: 'new' } }); } navigateToExternal() { this[NavigationMixin.Navigate]({ type: 'standard__webPage', attributes: { url: 'https://www.example.com' } }); } }
This sample code demonstrates a basic implementation where clicking a menu in your component will navigate different pages based on the selection.
Common Pitfalls and How to Avoid Them
- Overcomplicating your navigation: Keep it simple and intuitive.
- Neglecting mobile responsiveness: Ensure your navigation is just as effective on mobile devices.
- Ignoring accessibility standards: Make your application accessible to everyone, including those with disabilities.
Real-World Applications and Benefits
Custom Navigation can be a game-changer in applications that require guiding the user through a series of steps or pages, such as guided selling tools, customer-facing portals, and complex data entry forms. The benefits include improved user satisfaction, increased efficiency, and enhanced usability.
Conclusion
Implementing Custom Navigation in Lightning Web Components is not just about enhancing the visual appeal or technical robustness of your applications. It’s about creating a seamless, intuitive user experience that makes your applications delightful to use. By understanding the basics, leveraging the NavigationMixin, and applying best practices, you can create powerful, user-centric navigation solutions that drive success.
Next Steps and Additional Resources
- Experiment with more complex navigation patterns.
- Explore the Salesforce documentation for advanced features and capabilities.
- Connect with the Salesforce developer community for insights and support.
Start implementing custom navigation in your Lightning Web Components today and take your Salesforce applications to the next level!
More on Lightning Web Components
Salesforce Lightning Component Library
This guide provides a comprehensive overview and practical insights into mastering custom navigation within Lightning Web Components. By following the outlined steps, leveraging the power of NavigationMixin, and keeping in mind the common pitfalls, developers can significantly enhance the usability and functionality of their Salesforce applications.